In Laravel, the template engine is called Blade. It is a powerful, simple, and flexible templating engine that allows you to create dynamic views efficiently. Blade helps separate the logic of your application from the presentation layer, making your code cleaner and more maintainable.
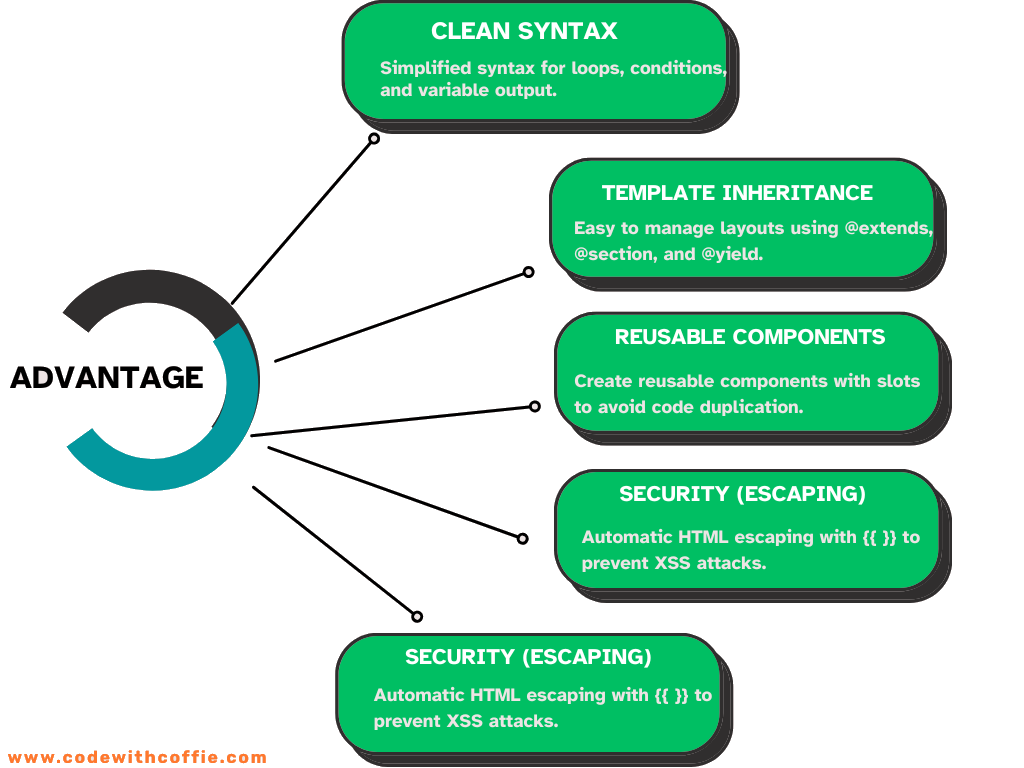
🔑 Key Features of Blade:
Lightweight & Fast:
Blade templates are compiled into plain PHP code and cached until they are modified. This makes them very fast, as there’s no overhead during rendering.
Template Inheritance:
You can create a master layout (like layout.blade.php
) and extend it in child templates. This helps maintain a consistent design across pages.
<!-- layout.blade.php -->
<html>
<head><title>@yield('title')</title></head>
<body>
<div class="container">
@yield('content')
</div>
</body>
</html>
<!-- home.blade.php -->
@extends('layout')
@section('title', 'Home Page')
@section('content')
<h1>Welcome to My Blog!</h1>
@endsection
Echoing Data:
You can display dynamic content using double curly braces {{ }}
which automatically escapes the output to prevent XSS attacks.
<h1>Hello, {{ $name }}!</h1>
if you want to output raw HTML (without escaping), you can use {!! !!}
:
{!! $htmlContent !!}
Control Structures:
Blade provides simple syntax for control structures like if
, for
, foreach
, and while
:
@if($user)
<p>Welcome, {{ $user->name }}!</p>
@else
<p>Guest User</p>
@endif
@foreach($posts as $post)
<h2>{{ $post->title }}</h2>
<p>{{ $post->content }}</p>
@endforeach
Components & Slots:
Reusable components can be created to keep your code DRY.
<!-- resources/views/components/alert.blade.php -->
<div class="alert alert-{{ $type }}">
{{ $slot }}
</div>
<x-alert type="success">Post saved successfully!</x-alert>
Custom Directives:
You can define your own Blade directives if needed:
Blade::directive('datetime', function ($expression) {
return "<?php echo ($expression)->format('m/d/Y H:i'); ?>";
});
Usage :
@datetime($post->created_at)
🚀 Why Use Blade in Laravel?
- Clean Syntax: Makes HTML templates look clean and readable.
- Efficient: Compiled into optimized PHP code.
- Security: Escapes output by default to prevent vulnerabilities.
- Reusability: Components, layouts, and directives promote DRY principles.