For n = 5
, the pattern looks like:
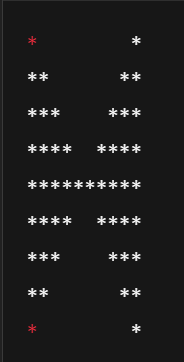
This has two parts:
- Upper Half – Rows from 1 to
n
- Lower Half – Rows from
n
to 1
🧠 Logic Behind It
For any row i
:
- Print
i
stars - Print
2 * (n - i)
spaces - Print
i
stars again
📝 Full PHP Code with Comments
<?php
$n = 5; // You can change this number for larger pattern
// Upper half of the butterfly
for ($i = 1; $i <= $n; $i++) {
// Left stars
for ($j = 1; $j <= $i; $j++) {
echo "*";
}
// Spaces
for ($j = 1; $j <= 2 * ($n - $i); $j++) {
echo " ";
}
// Right stars
for ($j = 1; $j <= $i; $j++) {
echo "*";
}
// New line
echo "\n";
}
// Lower half of the butterfly
for ($i = $n; $i >= 1; $i--) {
// Left stars
for ($j = 1; $j <= $i; $j++) {
echo "*";
}
// Spaces
for ($j = 1; $j <= 2 * ($n - $i); $j++) {
echo " ";
}
// Right stars
for ($j = 1; $j <= $i; $j++) {
echo "*";
}
// New line
echo "\n";
}
?>
▶️ How It Works
Hey guys! 👋 Today, we’re building a butterfly star pattern in PHP 🦋
👉 Step 1: We decide how many rows we want. Let’s say $n = 5
.
👉 Step 2: For the upper half, we loop from 1
to n
. Each row:
- Adds stars on the left (
$i
times) - Then spaces in the middle (
2 * (n - i)
times) - Then again stars on the right (
$i
times)
👉 Step 3: For the lower half, we just reverse it – loop from $n
down to 1
.
And voilà! You get this beautiful symmetrical butterfly.
Would you like the HTML version too for browser output? Or maybe a video script for a full YouTube-style tutorial? 😄